What is better?
Laravel, Drupal or WordPress?
There is no “better”, because they are completely different ecosystems.
Better question is what is more appropriate for yours specific use case?
This guide is for both tech and non-tech people.
Foreach (developer pun intended) ecosystem there are some benefits and downsides compared to the others on this list.
Let’s get started.
Laravel
What is Laravel? Laravel is a framework.
For a developer it gives more freedom to create whatever you want in a easy way.
Downsides of Laravel
No roles and permissions out of the box
Well since it is a framework and not a CMS there are no roles and permission built-in per se.
There is Laravel Sanctum or even the Spatie Permissions package that helps you with the database/code level of these things. But none of those have a GUI (graphical user interface) to manage them
No administration panel out of the box
This one is connected to the point above. Since using the framework is flexible and is intended to create just about anything an administration panel would be overkill. For developers, this just means more work. On another hand, the admin panel is fitted for the client perfectly then.
A fair note is that there is a plugin called October for some “generic” functionality.
No built-in translation GUI (Graphic User Interface)
While Laravel has a fairly simple and intuitive translation system:
<?php
#file resources/lang/app.php
return [
'hello_world' => 'Hello World'
];
#file app.blade.php
@lang('app.hello_world')
{{trans('app.hello_world')}}
It still lacks the user interface for a non-technical person to easily translate all of the strings to new languages.
Magic methods
Laravel uses the PHP magic methods to call things.
What are magic methods anyway?
Magic methods will never directly be called by the programmer – actually, PHP will call the method ‘behind the scenes. This is why they are called ‘magic’ methods – because they are never directly called, and they allow the programmer to do some pretty powerful things.
One of the best and commonly used examples of the magic methods are the __construct
and __get
magic method.
Here is an example of how magic methods are used
#fetch blog post by slug without and magic method
Blog::where('slug','my-blog-post')->first();
#fetch blog post by slug with an magic method
Blog::whereSlug('my-blog-post')->first();
Why is that bad?
Basically, you rely on methods you do not fully understand.
From the example above they are really simple to use and easy to read the downside is that they are slow, API’s declared by them are unclear.
Cutting edge
When an agency or a developer tries to sell you their services or they are trying to recruit you, this is often a perk.
Underline keeping Laravel up to date is a headache since it has a rapid deployment cycle.
We handled the downsides, what about the good things?
Benefits of Laravel
Laravel documentation / Laracasts.com
Looking at the Laravel official documentation you can see that the documentation itself is for both beginners and power users. Just easy to use and navigate.
In addition to the official documentation, there is the Laracasts website. At one point its the creator: Jeffrey Way, described it as “It’s like Netflix-for developers”. Jeffrey’s approach is so simple that anyone can follow easily.
Database migrations
If you ever built an custom web application one of the questions is: “How to export the database?”.
Laravel database migrations enable you to write all of your database manipulations in files, and then migrating them with one simple command.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateUsersTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->timestamp('email_verified_at')->nullable();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('users');
}
}
After that just run in your terminal
php artisan migrate
and voila!
Eloquent ORM
ORM sounds complex right? Object-Relational Mapping also. But it is fairly simple explanation.
ORM is an elegant way of querying the database data,but mapping them as an object . And if an ORM overall is an elegant way of writing queries, the Eloquent ORM is elegant query writing on stereoids.
Creating a model (along with controller factory and migration is easy.
php artisan make:model Blog -mfsc
Relationships are done fairly simply. In this example will will presume that We have an author model
public function author()
{
return $this->belongsTo(Author::class);
}
Here is a couple of eloquent commands to show how simple and intuitive Eloquent is
//finding and model by id
Blog::find(1);
//get all Blogs
Blog::all();
//get getting the relation author email
Blog::find(1)->author->email;
//Deleting the model
Blog::destroy(1);
Blade templates
Let’s compare a simple for each in plain PHP, Twig, and Blade
//plain php
<ul>
<?php foreach($user as $user): ?>
<li><?=$user->username;?></li>
<?php endforeach; ?>
</ul>
//twig
<ul>
{% for user in users %}
<li>{{ user.username|e }}</li>
{% endfor %}
</ul>
//blade
//plain php
<ul>
@foreach($user as $user)
<li>{{$user->username}}</li>
@endforeach
</ul>
Although twig is a more cross-domain syntax similar to javascript outputting, the opening tags are completely unintuitive. Blade is completely intuitive to the PHP world and has far simpler syntax in opposed to plain PHP.
Plays great with Vue
This point is straight underneath the Blade templates since they are connected. It plays perfectly with Vue and any other Front-end framework for that matter. React, Angular you name it.
It is easy to set it up as 1,2,3
//install vue via console
npm install --save vue@next && npm install --save-dev vue-loader@next
Then add it to your webpack.mix
mix.js('resources/js/app.js', 'public/js').postCss('resources/css/app.css', 'public/css', [])
.vue();
Last, create your component and load it in the app.js
import { createApp } from 'vue'
import MyLaravelWorld from './components/MyLaravelWorld.vue';
const app = createApp({});
app.component('hello-world', MyLaravelWorld)
.mount('#app');
require('./bootstrap');
Collections
Collections
Collections are array wrappers. Does not seem that much right?
They offer us manipulation of arrays but in a object style. In addition to easier code writing (no need to write the brackets to access the key), you can also chain your methods
Example: I want to take all of the value keys from the subarray in a separate variable and I want to uppercase them.
$array = [['field_name' => 'Marijan', 'value' => 'mid'],['field_name' => 'Gudelj', 'value' => 'senior'] ];
//usual way
$plainArray = array_column($array, 'value');
foreach($plainArray as $key => $v){
$plainArray[$key] = strtoupper($v);
}
//Laravel
//collect transforms array to collection
$laravelCollection = collect($array)->pluck('value')->map(function ($name) {
return strtoupper($name);
});
The Laravel result
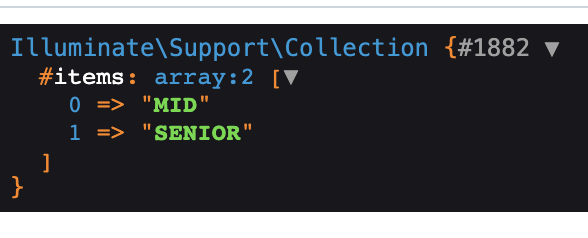
Laravel official packages
Laravel tries to keep stuff as clean and lightweight as possible. Not only for installation processes but also for the Laravel services container. That is why all of the plugins are optional but easy to add.
We have Laravel Valet or Homestead Server, Sanctum for SPA/mobile/token-based authentication,
Passport for authentication with OAuth2, Scout for driver-based full-text search, Cashier for payments, and many more.
– Sanctum
– Scout
– Passport
– Cashier
– Socialite
– Dusk
Using the latest technologies available
Since Laravel is a cutting-edge framework it always uses the latest PHP versions available, follows the latest frontend technologies trends as well. The result is that it always in a way enforces you to be the best you can be as a developer.
Community
It is one of the largest growing frameworks you can find support and questions on both Stackoverflow and Laracasts forum. In addition to that, due to it’s age and popularity there is a lot of plugins on github.
When to use Laravel
Laravel should be used when you have custom applications where you need as much freedom to create your application and fresh ideas, and a lot of database manipulations.
Also due to Eloquent Lumen(a lightweight version of Laravel) is perfect for API building. If you need something more generic like a Blog or a simple site- Laravel is not a way to go.
WordPress
WordPress. The CMS (Content Managment System) that rules the web. Or in 2021 over 455 million (455 000 000 ) websites use WordPress. That is not a small number.
This is a that was initially conceived as a blog site. Over the years it has became the “go to” as a website solution for any idea. This gives us an broad spectrum of plugins and themes available for rapid website building.
Downside of WordPress
Often targeted by hackers
A so big system is uncontrolled. Poor developing and a lot of users is a perfect target for injecting an malicious code, leaving the website owners with a huge cleanup bill or loss of data.
Often the plugin/theme is poor
A lot od developers place their plugins online to either get the fame and glory or to earn some money. That is fine.
Current number of WordPress plugins is 57,994.
But nobody controls how the plugins are developed, there are no obivoys guidelines. Only the method if it works or does not work. Drupal for example has an notice if the developer is not in the security opt in program, and that you use the module at your own risk. In addition to that, to opt into the programme you need to know all of the Drupal guidelines. This is something that WordPress lacks.
No built-in speed optimization system
Drupal has an included CSS or JS modifications and concatenation settings in it’s admin panel, in Laravel we have Laravel webmix that is an webpack wrapper (and have full control over our assets).
WordPress on the other hand has nothing. You need to install one or more plugins or do a lot of manual work to optimize it and have a great SEO score.
Website speed
We have covered plugins and assets. One of the Worpdresses problems is the database bottleneck and the way the plugins are loaded.
WordPress site will still be slow down even if your plugin is disabled. Needless to say having a large number of plugins slows down everything. Add the Heartbeat, no built in speed optimization and cheap hostings into the calculations the end result is an disaster. It is really hard to go “in the green” on Google page speed insights.
The 1s mark can rarely be achived.
No built in translation system GUI (Graphic User Interface) and language switcher
Same as Laravel, while it has an standardised way of translating this over the .po/.mo files there is no user interface to do it. Unless you use a plugin. Also for language switching the most often solution is to use the WPML plugin -that does not come in cheap.
A lot of developers
For developers a lot of WordPress developers means that is a bit harder to find high quality jobs. On the other hand the employers can take their pick. Somtimes it is just an matter of trying until you find the right client-dev “click”.
Benefits of WordPress
Themes and plugins
A lot of both free and paid plugins and themes are available. Maybe the client has his heart set on some functionality or theme? There is a high chance that exactly that feature is a WordPress feature.
This shortens te website development time very very fast. Just check templateforest.net or Envato elements.
Rapid development
Compared to Drupal, WordPress does not have a high learning curve. That means that there is a lot of WordPress developers out there. And the great developers can alone build the sites and features really fast. But if you need a hand, or two for that matter you can find a WordPress developer without breaking a sweat.
Roles and permissions
Compared to Laravel, WordPress offers Roles and permissions out of the box.
Sure you have the subscriber, author, editor, administrator or in certain cases the super admin role (multisite feature).
Sure you can create new user roles with the administrator role.
Compared to Drupal’s Role and permissions system it can be considered as a basic system.
The reason for that is that if you want to customize the user role you need to use a plugin.
Administration interface
Unlike Laravel you have an administrator interface out of the box. In addition to that, the interface itself has better visibility compared to Drupal’s admin interface. We are talking about out-of-the-box features. WIth Drupal, you can change the admin theme, but more on that later on.
WooCommerce (for smaller webshops)
One of the absolute winners of WordPress is the Woocommerce plugin. It can cover shops up to 1000 orders per day (but the plugin count must be reasonable or very well optimized).
Anything beyond that is not recommended due to its database hierarchy and memory-eating capabilities. WooCommerces has a preferred memory limit of 768MB.
But if you need a shop with one vendor, that covers products and product variations, has a lot of payment gateways and you are on a limited or maybe even a mid-ranged budget WooCommerce is the way to go.
When to use WordPress?
Taking into consideration the hierarchy of WordPress it is a pretty straightforward option for basic company/brand sites, blog sites or WooCommerce sites (up to 1000 orders per day). Anything out of this range will be stretching its capabilities and you will have to inject a huge amount of money to do the periodical speed optimization.
Drupal
Drupal is not a framework, it is not a CMS, it is a CMF (Content Management Framework). Basically, it is a hybrid between Laravel and WordPress. That is why it is placed last on this list.
From all non-Drupal developers and even non-tech-savvy people, Drupal seems like an outdated system.
The reality is completely different. It is a fully scalable, bulky system.
There is the infamous “Drupal learning curve” or where the Drupal learning is exponentially hard.
But when you get the hang of how the Drupal documentation is “organized” it is a lot easier to learn.
From the developer’s perspective, the developers need to understand OOP and this is a major setback for a lot developers.
Drupal downsides
Dry learning sources
Documentation while being detailed, is fairly dry compared to Laravel’s friendly documentation system. Maybe a better explanation of the documentation itself is that is more like API documentation, missing the user-friendly versions.
Drupalize.me as a visual learning tool has just started to look a modern learning tool, especially comparing it to Laracasts.
Developers need to know Object Oriented Programming (OOP) really good
This is a good and bad side.
All programmers know how to create a class. But do they really know the difference between an interface or an abstract class? Why and when to use private and public functions?
We will cover all of these questions in another blog post.
But compared to WordPress the developers need to know their stuff. The bad side is that you need to find a developer (and he will not come cheap). On the other side, the site quality should be significantly higher-at least in theory.
Code formatting
Drupal enforces a kind of modified PSR-1 formatting. As PHP is concerned PSR-2 is becoming the most common coding standard.
Here is an example. Drupal uses 2 space indents, while PSR-2 uses four spaces. Drupal’s opening bracket in a method is in the same line, while the PSR-2 is in the line underneath.
//Drupal
namespace Vendor\Package;
use FooInterface;
use BarClass as Bar;
use OtherVendor\OtherPackage\BazClass;
class Foo extends Bar implements FooInterface {
final public static function bar() {
// method body
}
public function sampleMethod($a, $b = null) {
if ($a === $b) {
bar();
} elseif ($a > $b) {
$foo->bar($arg1);
} else {
BazClass::bar($arg2, $arg3);
}
}
}
//PSR-2
namespace Vendor\Package;
use FooInterface;
use BarClass as Bar;
use OtherVendor\OtherPackage\BazClass;
class Foo extends Bar implements FooInterface
{
public function sampleMethod($a, $b = null)
{
if ($a === $b) {
bar();
} elseif ($a > $b) {
$foo->bar($arg1);
} else {
BazClass::bar($arg2, $arg3);
}
}
final public static function bar()
{
// method body
}
}
PSR-2 is more intuitive to read, especially if you have a lot of brackets on your hands.
Modules
Comparing to WordPress plugins and Laravel packages, one of the Drupal benefits (Community) can be it’s weak point. In the Drupal community, there is not duplicate module. Module may have the same names, but the functionality needs to be different from all existing similar modules. Now this has is great sides, but from a perspective of rapid development, and amplified by the Drupal community pool size it will probably prolongue your developemnt time, since, compared to WordPress there is an high chance that there isn’t module suited for your needs.
Drupal benefits
- Highly scalable
- Role and permissions of ouf the box that are easy to implement everywhere
- Admin interface out of the box
- Four ways of building the layout out of the box( Layout builder,Blocks,Drupal views and Twig templates)
- Layout builder itself
- Translations out of the box
- Defining your content type (similar to WordPress custom post type but more flexible)
- Configurations for seamless migrations between local,dev,production environment
- Robust API
- Out of the box asset optimization
- For large webshops Drupal Commerce
- Community
When to use Drupal?
When you have a website that can easy become a large website, When you need a website that you need reusable components. Use it when the code is not fully custom. It has it’s benefits in front of WordPress because it has the translation system. In addition to that the layout builder gives you a lot of options as an user to build your own layouts.
Conclusion
There you go ladies and gentleman. This is a vague overview of all of all three different systems. Whatever you select it is important to have an vision for your page/app and the costs will be really reasonable.